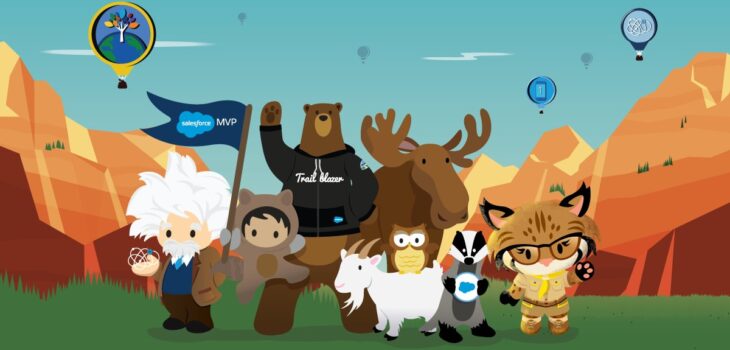
Calling the REST API from LWC
This article shows how to call REST endpoints from lightning web components.
The following example will get the IP Address of the current user and callout to get country code from third party service.
Component HTML:
<template> <p if:true={userIpAdress}> IP ADDRESS : {userIpAdress} </p> <p if:true={userCountryCode}> COUNTRY CODE : {userCountryCode} </p> </template>
Hook your Apex Method to your JS file using wire methods.
import { LightningElement, track, wire } from 'lwc'; import getIpAddress from '@salesforce/apex/ApexClass.getIpAddress'; export default class IpDetails extends LightningElement { @track userIpAdress;
@track userCountryCode; @wire(getIpDetails)ipAddress({data, error}){ if(data) { this.userIpAdress = data.ip; this.userCountryCode = data.country_code; } else if (error) { this.error = error; this.userIpAdress = undefined; } }; }
APEX
@AuraEnabled(cacheable=true) public static Map<String,String> getIpAddress() { String ip = Auth.SessionManagement.getCurrentSession().get('SourceIp'); String countryCode = getCountryCodesFromIp(ip); Map<String,String> output = new Map<String,String>{ 'ip' => ip, 'country_code' => getCountryCodesFromIp(ip) }; return output; } /*** * API CALL TO GET COUNTRY CODE FROM IP ADDRESS */ public static String getCountryCodesFromIp(String userIPAddress){ String countryCode; Http httpProtocol = new Http(); HttpRequest request = new HttpRequest(); //USING NAMED CREDENTIALS String endpoint = 'callout:IPCountry_Host/'+userIPAddress+'?access_key='+ GET_YOUR_API_KEY; request.setEndPoint(endpoint); request.setMethod('GET'); HttpResponse res = httpProtocol.send(request); System.debug('response :'+res.getBody() ); String responseText = res.getBody(); // parse response Map<String, Object> responseObject = (Map<String, Object>)System.JSON.deserializeUntyped(responseText); if((String)responseObject.get('country_code') != '') { countryCode = (String)responseObject.get('country_code'); } return countryCode; }
Calling REST Api from Apex will probably be the safest and preferred way to perform callouts.
Having said that, in some cases it is useful to use client side callouts – The example below shows how to get your IP address using a XMLHttpRequest
.
Here we don’t use Apex and all the magic happens from the JS code – just make sure you white list the endpoint on CSP Site. (Setup > CSP).
connectedCallback(){ // calling the method this.userIpAdressClient = this.getPublicIPAddress(); console.log('ip ' + this.userIpAdressClient); } // example for client side callout getPublicIPAddress(){ let xmlhttp; if (window.XMLHttpRequest) xmlhttp = new XMLHttpRequest(); else xmlhttp = new ActiveXObject("Microsoft.XMLHTTP"); // ** TODO: white list CSP Sites xmlhttp.open("GET","https://api.ipify.org?format=jsonp=",false); xmlhttp.send(); let hostipInfo = xmlhttp.responseText; if(hostipInfo) { return hostipInfo; } return false; }