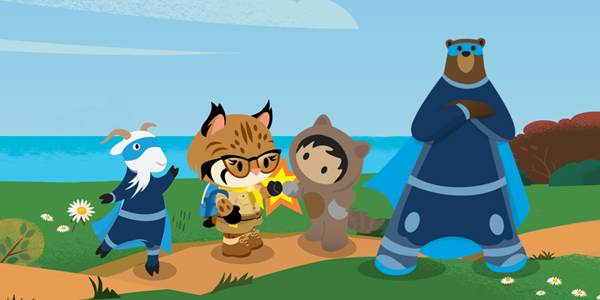
Using Schema classes to build useful Utility Metadata methods
The following examples shows useful describe methods using the Schema class.
This helps to build a Utility Class or methods where we can re-use across different functionality.
Discover Object Name from Object Id
- Give me the Record Id and I will tell you the object Name
public static String getObjectFromId(Id contextId) { String objectName = contextId.getSObjectType().getDescribe().getName(); return objectName; }
Working with Fields
- The following example shows how to get all the fields from a given sObjectName or simply from a record Id.
- This can help to build a form or to allow some kind of field selection to update by a user on the UI.
Describe Field Result
For each field member there are some useful describe result methods which allows to check the Type of the field or get the attributes from the given field. (Label, Required, Encrypted, Type…)
public static String getAllFieldsFromId( Id contextId ) { List <Map<String,Object>> fieldsList = new List <Map<String,Object>> (); Map<String,Object> data = new Map<String,Object>(); String objectName = contextId.getSObjectType().getDescribe().getName(); Schema.SObjectType mySObj = Schema.getGlobalDescribe().get(objectName); // Get sObject Describe Result Schema.DescribeSObjectResult mySObjDescribe = mySObj.getDescribe(); // Get Field Map Map<String,Schema.SObjectField> mapFields = mySObjDescribe.fields.getMap(); for(String fieldName : mapFields.keyset()){ Schema.DescribeFieldResult fieldDescribe = mapFields.get(fieldName).getDescribe(); Map<String,Object> fieldMap = new Map<String,Object>{ 'FieldAPIName__c' => fieldDescribe.getName(), 'Field' => fieldDescribe.getLabel(), 'Type' => fieldDescribe.getType(), 'isAccessible' => fieldDescribe.isAccessible(), 'isCustom' => fieldDescribe.isCustom() }; fieldsList.add(fieldMap); } data.put('allFields',fieldsList); data.put('totalCount',mapFields.keyset().size()); return JSON.serialize(data); }
Describe and Get Field Type
public static String getFieldType ( String objectName, String fieldApiName ) { Map<String,Schema.SObjectField> mapFields =Schema.getGlobalDescribe().get(objectName).getDescribe().fields.getMap(); Schema.DescribeFieldResult fieldDescribe =mapFields.get(fieldApiName).getDescribe(); String fieldType = (String)String.valueOf(fieldDescribe.getType()); return fieldType; }
Get Only Fields from a specific Type (eg. Lookup Fields – Reference)
public static Map<String,Object> getLookupsFromObject( String objectName ) { Map<String,Object> data = new Map<String,Object>(); Schema.SObjectType mySObj = Schema.getGlobalDescribe().get(objectName); // Get sObject Describe Result Schema.DescribeSObjectResult mySObjDescribe = mySObj.getDescribe(); // Get Field Map Map<String,Schema.SObjectField> mapFields = mySObjDescribe.fields.getMap(); for(String fieldName : mapFields.keyset()){ Schema.DescribeFieldResult fieldDescribe = mapFields.get(fieldName).getDescribe(); if(fieldDescribe.getType() === Schema.DisplayType.Reference) { data.put(fieldName, fieldDescribe.getLabel()); } } return data; }
Describe and Get All Schema Objects
- This example shows how to get some common attributes from a schema sObject.
- Getting the object
isUpdateable
,isCreateable
can help validate DML operation before execution.
public static String getAllSchemaObjects() { List <Map<String,Object>> objectList = new List <Map<String,Object>> (); Map<String,Object> data = new Map<String,Object>(); Map<String, Schema.SObjectType> allObjects = Schema.getGlobalDescribe(); for(String objectName : allObjects.keyset()){ Schema.DescribeSObjectResult objectTypeDescribe = allObjects.get(objectName).getDescribe(); Map<String,Object> objectMap = new Map<String,Object>{ 'apiName' => objectTypeDescribe.getName(), 'label' => objectTypeDescribe.getLabel(), 'pluralLabel' => objectTypeDescribe.getLabelPlural(), 'prefix' => objectTypeDescribe.getKeyPrefix(), 'isCustom' => objectTypeDescribe.isCustom(), 'isAccessible' => objectTypeDescribe.isAccessible(), 'isCreateable' => objectTypeDescribe.isCreateable(), 'isDeletable' => objectTypeDescribe.isDeletable(), 'isQueryable' => objectTypeDescribe.isQueryable(), 'isSearchable' => objectTypeDescribe.isSearchable(), 'isUpdateable' => objectTypeDescribe.isUpdateable() }; objectList.add(objectMap); } data.put('allObjects',objectList); return JSON.serialize(data); }
Get Child Related Lists from a given sObject name
- Shows how to use some useful methods on Schema.ChildRelationship
public static String getRelatedListsFromObject( String objectName ) { List <Map<String,Object>> fieldList = new List <Map<String,Object>> (); Map<String,Object> data = new Map<String,Object>(); Schema.SObjectType mySObj = Schema.getGlobalDescribe().get(objectName); // Get sObject Describe Result Schema.DescribeSObjectResult related = mySObj.getDescribe(); for (Schema.ChildRelationship cr: related.getChildRelationships() { system.debug('====child object==='+cr.getChildSObject()); Map<String,Object> fieldMap = new Map<String,Object>{ 'key' => cr.getChildSObject(), 'field' => cr.getField(), 'name' => cr.getRelationshipName() }; fieldList.add(fieldMap); } data.put('related',fieldList); return JSON.serialize(data); }