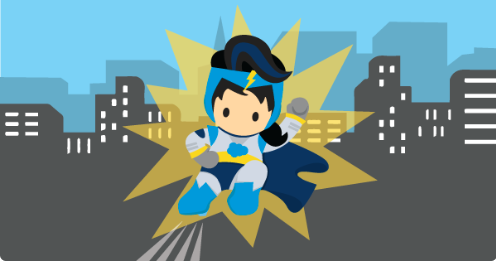
Loading Static Resource into a Lightning Component
This article will show how to use a Static Resource in Lightning components. As in many cases we need to import resources – images, css, js etc.. into our application.
To get started we will need to upload our images and digital assets as a Static resource in our Salesforce instance.
Upload Static Resource
Upload Static Resource from Setup UI :
- Setup > Static Resources >New
There you will give the resource a name and description and mark the resource as private/public to control the access to the resources and how it will be cached in browser.
Cache Control :
- Private : The static resource is only stored in cache for the current user’s session (shouldn’t be shared with other users).
- Public : specifies that the static resource data cached on the Salesforce server be shared with other users in your organization for faster load times.
Upload Static Resource from your IDE :
1. Create XML file named – myResource.resource-meta.xml
- Copy and Paste the following :
<?xml version="1.0" encoding="UTF-8" ?> <StaticResource xmlns="http://soap.sforce.com/2006/04/metadata"> <cacheControl>Public</cacheControl> <contentType>application/zip</contentType> </StaticResource>
2. Create a Matching folder named ‘myResource’ and place your files inside.
- ./myResource
- style.css
- core.js
- ./myResource.resource-meta.xml
3. Deploy/Push your code to your org
sfdx force:source:push -u
Referencing Static Resources in Lightning Components
LWC Examples
Loading custom or third party CSS or JS files
import { LightningElement } from "lwc"; import resourceName from "@salesforce/resourceUrl/resourceName"; import { loadScript, loadStyle } from "lightning/platformResourceLoader"; export default class MyComponent extends LightningElement { isInitialized = false; renderedCallback() { if (this.isInitialized) { return; } this.isInitialized = true; Promise.all([ //loading the js loadScript(this, resourceName + "/lib/core.js"), // loading css loadStyle(this, resourceName + "/lib/style.css") ]) .then(() => { console.log("resources loaded successfully "); this.doStuff(); }) .catch(error => { console.log("failed to load resources"); }); } }
loadScript
andloadStyle
methods can help us load the resources asynchronously and do some stuff after it has been loaded.
Display Images from your resource
// JS import resourceName from '@salesforce/resourceUrl/resourceName'; export default class MyComponent extends LightningElement { myImage = resourceName + '/images/myImage.svg'; }
<!-- HTML --> <img src={myImage} class="slds-align_absolute-center"></img>
Aura
Require a $Resource
in Aura .cmp file
<!-- myComponent.cmp --> <aura:component> <ltng:require styles="{!$Resource.myResource + '/assets/styles/style.css'}" scripts="{!$Resource. myResource + '/core.js'}" afterScriptsLoaded="{!c.scriptsLoaded}" /> </aura:component>
// myComponentController.js scriptsLoaded: function(component, event, helper) { console.log('resources loaded successfully'); }
Using $Resource
in JavaScript for aura
const myImage = $A.get('$Resource.yourGraphics') + '/images/avatar1.jpg';