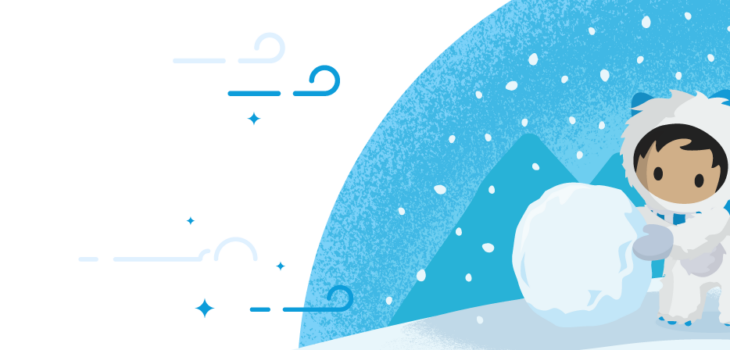
LWC OSS – Open Source – Setting up
Lightning Web component can be used as a standalone framework/app which can work outside of Salesforce platform as well.
Like any other Web framework tool (eg. react, Angular etc..), We can now use LWC as a complete framework for our Website/Desktop app as JS engine, UI component framework and bundling.
We can quickly scaffold a Local Web project in our CLI and deploy to any Domain or host.
- Official Documentation – LWC Open Source System (OSS)
Use the command below to create the basic project structure with the name which can be change my-app
npx create-lwc-app my-app
- We will be changing the files so go with the basic setup structure and follow the steps to see the configuration available.
CLI Command Process
It will ask a few questions on your terminal to tailor the setup to your needs.
- Do you want to use the simple setup?
- Yes
- Package name for npm?
- We can just leave it the default by pressing Enter key
- Select the type of app you want to create
- Standard web app
- Progressive Web App (PWA)
- Electron app
- Do you want a basic Express API server?
- Yes
Once this process has completed – Open your new project folder in VS Code IDE.
- Run
code my-app
You may already open your terminal and run the command to preview your skeleton LWC app on your browser:
npm run watch
- This will run on your new local server on port
http://localhost:3001/
and can be access via your browser. - Start your journey by looking at your
package.json
to understand which dependencies has already been install as initial setup and what can you use to setup your app with all goodies.
Adding Lightning Base Component with SLDS to your project
The lwc-services
configuration would allow to build your public compiled folder of your project.
- We will write it to do the Following (It’s like webpack configuration…):
- Transfer the SLDS assets folder into our Resources folder
- Build our `dist` public minified folder
- First Let’s install Salesforce Lightning Design System (SLDS)
npm install @salesforce-ux/design-system
- You should install the
synthetic-shadow
package and thelightning-base-components
- Base components are powerful to get started quickly and they require a virtual dom.
npm install @lwc/synthetic-shadow
npm install lightning-base-components
Lets setup the build process so it will include the correct references for folder structure and SLDS
lwc-services.config.js
// Find the full example of all available configuration options at // https://github.com/muenzpraeger/create-lwc-app/blob/main/packages/lwc-services/example/lwc-services.config.js const buildFolder = './dist'; const srcFolder = 'src/client'; module.exports = { buildDir: `${buildFolder}`, sourceDir: `./${srcFolder}`, resources: [ { from: `${srcFolder}/resources/`, to: `${buildFolder}/resources/` }, { from: 'node_modules/@salesforce-ux/design-system/assets', to: `${srcFolder}/assets` }, { from: `${srcFolder}/assets/`, to: `${buildFolder}/assets/` }, ], devServer: { proxy: { '/': 'http://localhost:5000' } } };
- visit the
lwc.config.json
file in order to references the modules folder along with the base components
{ "modules": [ { "dir": "src/client/modules" }, { "npm": "lightning-base-components" } ] }
Run your build script to include the SLDS resource in your project
npm run build:development
Setup your Express Server
In order to setup API routes and serving your app we can go to our server file – main.js
.
- This is where we can configure the single page app and URL path that user can or cannot access.
- We basically telling the server to always serve our
index.html
file on any*
path on our domain. (Thats the file that serves our LWC app)
const express = require('express'); const compression = require('compression'); const helmet = require('helmet'); const path = require('path'); const app = express(); app.use(helmet(), compression(), express.json()); const HOST = process.env.HOST || 'localhost'; const PORT = process.env.PORT || 5000; const SERVER_URL = `http://${HOST}:${PORT}`; // const DIST_DIR = './dist'; const DIST_DIR = './src/client'; app.use(express.static(DIST_DIR)); app.use('*', (req, res) => { res.sendFile(path.resolve(DIST_DIR, 'index.html')); }); app.listen(PORT, () => console.log(`✅ API Server started: ${SERVER_URL}`));
Bring it all together on your Client App
Setup your index.html
page and inject the container LWC as a dom element.
- Our
index.html
holds the main app that is being appended(injected) into the page.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8" /> <title>YV Start Kit - LWC OSS</title> <link rel="stylesheet" type="text/css" href="./assets/styles/salesforce-lightning-design-system.min.css" /> <!-- Basic Styling --> <link rel="stylesheet" href="./resources/style/main.css" /> <!-- Block zoom --> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1" /> <link rel="shortcut icon" href="./resources/favicon.ico" /> </head> <body> <div id="main"></div> </body> </html>
index.js
– will also import thesynthetic-shadow
- Reference your container app from the modules folder and append it on the DOM.
import '@lwc/synthetic-shadow'; import { createElement } from 'lwc'; import MyApp from 'my/app'; const app = createElement('my-app', { is: MyApp }); // eslint-disable-next-line @lwc/lwc/no-document-query document.querySelector('#main').appendChild(app);
This is your main LWC container app – which can hold any child component inside it – Feel free to start playing with your components and build some initial UI to play with.
The Next thing you probably would want to setup is our actual server and some kind of a Router so a User can navigate between different pages on your App. – Next Article will cover this deeply.
For now :
- Develop and Watch changes in real time – simply run:
npm run watch
- To build/compile all into the
dist
folder that can be deployed to Heroku or any web hosting solution.
npm run build
Start Building your components
Let’s start mess around with our LWC modules app.html
and start build components.
<template> <div class="slds-grid slds-grid_vertical slds-grid_vertical-align-center "> <lightning-button label="Button1" name="mainButton" variant="brand" onclick={handleClick}></lightning-button> <lightning-button label="Button2" name="otherButton" variant="success" onclick={handleClick}></lightning-button> </div> </template>
I think that as next step Ill start playing with Navigation inside the components so an actual website will be possible. – Visit Here
Upload your App to Heroku and make it Public
If we wish to upload this project to Heroku we could simply create and account and do the following :
- Add a
Procfile
with the following run code
web: yarn serve
- Make sure your package.json scripts contain this command and that its pointing to the correct path.
"serve": "node src/server/main.js",
- Once pushed into a git repo or following the steps and creating an Heroku repo – deploy and you are good to go.
git push heroku
Next Steps
Once you feel comfortable to move into getting data from Salesforce – click here and continue reading