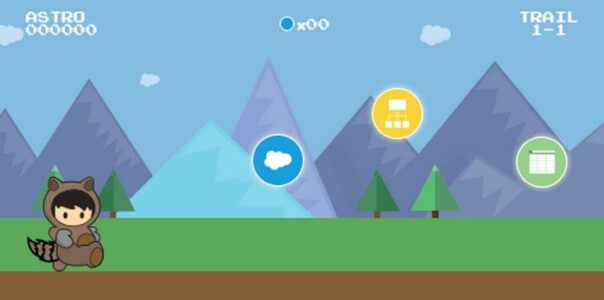
Controlling the finish behavior of lightning flow
Lightning flow allows to perform pretty complex business processes in a very configurable manner.
A smart combination of a process builder with a lightning flow can create some powerful automation managed by admins.
One common requirement though is to navigate somewhere after the flow has finished.
For some reason, I couldn’t find a proper way to do this in a configurable manner. So I had to resort to a custom component to the rescue.
In the below example I’ll show how to wrap our lightning flow with a component to control the finish behavior.
Create Aura Component
- Start By adding the
lightning:flow
component to your .cmp file - We also adding here a variable for the
flowName
and therecordId
we want to pass into the flow
<aura:component implements="force:hasRecordId" access="global"> <aura:attribute name="flowName" type="String" access="global" /> <aura:attribute name="recordId" type="String" access="global" /> <lightning:flow aura:id="flowData" onstatuschange="{!c.handleStatusChange}" /> </aura:component>
Invoke the Flow
onInit
call your JS controller `cmpTestController.js` :
doInit : function (component, event, helper) { let flow = component.find("flowData"); let flowName = component.get('v.flowName'); flow.startFlow(flowName); }
This will invoke your flow by it’s name that i this case declared as a design attribute.
Pass Parameters into a Flow
The startFlow
method can accept some input parameters and inject them to your flow:
let inputVariables = [ { name :"recordId", type :"String", value:component.get('v.recordId') } ]; flow.startFlow(flowName, inputVariables);
The Finish Behavior
When the flow finished its process it will change it’s status and invoke another JS callback function in your JS controller which allow you to finish the process with a certain action.
* For example – navigating into a different record.
Statuses to remember :
FINISHED_SCREEN
=> Autolaunched (Headless) FlowFINISHED
=> Screen based flow
Example :
handleStatusChange :function (component, event, helper) { let redirectOutput=component.get('v.redirectVar'); // redirect variable that holds the recordId to navigate to if( (event.getParam("status") === "FINISHED_SCREEN"||event.getParam("status") ==="FINISHED" ) ) { let outputVariables = event.getParam("outputVariables"); let outputVar; for(var i=0; i<outputVariables.length; i++) { outputVar=outputVariables[i]; if(outputVar.name===redirectOutput) { let urlEvent=$A.get("e.force:navigateToSObject"); urlEvent.setParams({ "recordId":outputVar.value, "isredirect":"true" }); urlEvent.fire(); } } } }