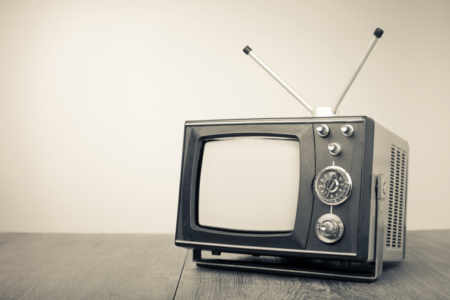
Embed a Visualforce page inside a component
This post will show a way to display or embed a Visualforce page inside a component.
Main use case I saw for this was supporting legacy Visualforce pages.
As of this post only way I found for doing this was using an iframe
tag with dynamic attributes inside the component.
Build the aura wrapper component
<aura:component controller="VFCompController" implements="flexipage:availableForAllPageTypes,force:hasRecordId"> <aura:attribute name="recordId" type="String" access="public" /> <aura:attribute name="vfHost" type="String" access="public" /> <aura:attribute name="pageName" type="String" access="public" /> <aura:attribute name="pageHeight" type="Integer" access="public" /> <div class="slds-grid"> <iframe aura:id="vfFrame" src="{!'https://' + v.vfHost + '/apex/'+ v.pageName}" height="{!v.pageHeight + 'px'}" class="view-frame"/> </div> </aura:component>
Getting your host domain url
- Apex has some built-in methods to retrieve the BaseUrl
- On your Apex controller “VFCompController” add the following
private static String baseUrl = URL.getSalesforceBaseUrl().getHost();
- populate the
vfHost
attribute with the value
Communication between the Component to your Visualforce
- To Communicate between the component to visualforce page we can use Events.
Send a Message From Component to Visualforce
({ sendToVF : function(component, event, helper) { let message = component.get("v.message"); let vfOrigin = "https://" + component.get("v.vfHost"); let vfWindow = component.find("vfFrame").getElement().contentWindow; vfWindow.postMessage(message, vfOrigin); } })
// On Visual force script var baseURL = "{!$CurrentPage.URL}"; var lexOrigin = "https://yourdomain-dev-ed.lightning.force.com"; window.addEventListener("message", function(event) { if (event.origin !== lexOrigin) { // Not the expected origin: reject message! return; } // Handle message console.log(event.data); }, false);
Send a Message From Visualforce to Lightning component
<!-- Get Session from browser and display --> <input style="display:none" id="message" type="text" value="{!$Api.Session_ID}"/> <!-- Send the Message --> <button onclick="sendToLC()">
Inside your <script>
tag add the following :
var baseURL = "{!$CurrentPage.URL}"; baseURL = baseURL.replace("--c.cs86.visual.force.com/apex/ncSessionGetter", ".lightning.force.com"); //var lexOrigin = "https://efficiency-site-4483-dev-ed.lightning.force.com"; function sendToLC() { console.log('vf says ' + baseURL); var message = document.getElementById("message").value; parent.postMessage(message, baseURL); }
Receiving the Message in a Lightning Component
doInit : function(component) { var vfOrigin = "https://" + component.get("v.vfHost"); // our listener window.addEventListener("message", function(event) { if (event.origin !== vfOrigin) { // Not the expected origin: Reject the message! return; } // Handle the message console.log(event.data); }, false); }
*
Note
note that if visualforce page is embedded in a community context base URL will change.
Documentation :