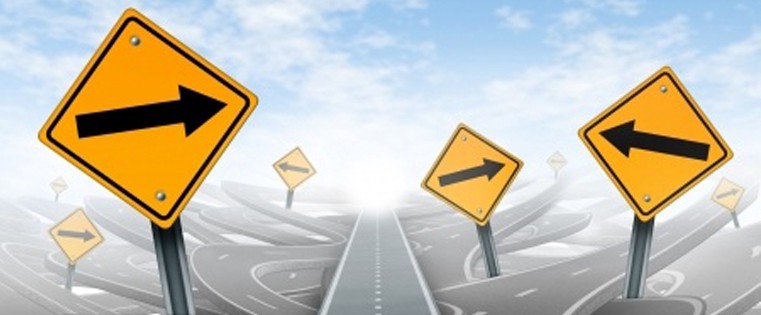
How to use Navigation service in Lightning
In order to control the navigation between pages and components Salesforce introduced the lightning navigation module. In this article we will explorer the way to use it both in Aura and LWC.
LWC
In Lightning web components we can use the built in module NavigationMixin
it can help us to generate a URL or navigate to a page reference object we define.
import { LightningElement} from 'lwc'; import { NavigationMixin } from 'lightning/navigation'; export default class NavigationExample extends NavigationMixin(LightningElement) { // navigation function navToURL(event){ event.preventDefault(); const urlPath = 'https://vardi.xyz'; const pageRef = { "type": "standard__webPage", "attributes": { "url": urlPath } } this[NavigationMixin.Navigate](pageRef); } // Generate a URL to a User record page generateURL(){ this[NavigationMixin.GenerateUrl]({ type: 'standard__recordPage', attributes: { recordId: '005B0000001ptf1IAE', actionName: 'view', }, }).then(url => { this.recordPageUrl = url; }); } }
Aura
Let’s start by adding to our component .cmp file the lightning:navigation
– this will allow to navigate to a given pageReference or to generate a URL from a pageReference.
<aura:component implements="flexipage:availableForAllPageTypes,forceCommunity:availableForAllPageTypes,force:hasRecordId,force:hasSObjectName" access="global" > <lightning:navigation aura:id="navigationService" /> </aura:component>
Then from your JS controller or helper files get it like this :
handleNavigateClick: function(component, event, helper) { const navService = component.find("navigationService"); const pageReference = { type: 'standard__objectPage', attributes: { objectApiName: 'Account', actionName: 'list' }, state: { filterName: "All" } }; event.preventDefault(); navService.navigate(pageReference); },
Each pageReference can be invoke using the following line
// will navigate to page reference name navService.navigate(pageReference);
Set a pageReference Object
To Navigate to an sObject Record Home :
const recordHomeReference = { type: 'standard__objectPage', attributes: { objectApiName: 'Account', actionName: 'home' } }; // will navigate to object home page navService.navigate(recordHomeReference);
Navigate to a Record Page :
const recordPageReference = { "type": "standard__recordPage", "attributes": { "recordId": component.('v.recordId'), "objectApiName": 'Account', "actionName": 'view' // clone, edit, or view } };
Navigate to a List View page :
- Add a state object with the List View Filter Name.
const listViewReference = { type: 'standard__objectPage', attributes: { objectApiName: "Account" , actionName: 'list' }, state: { "filterName": "ListViewName" } };
Navigate to a Related Child Object :
const relatedChildReference = { "type": "standard__recordRelationshipPage", "attributes": { "recordId": "PARENTID => AccountId", "objectApiName": "PARENT OBJECT NAME eg. ACCOUNT", "relationshipApiName": "TARGET CHILD RELATIONSHIP NAME", "actionName": 'view' } }
Navigate to a Lightning App Page :
const appPageReference = { "type": "standard__namedPage", "attributes": { "pageName": "APPNAME", } };