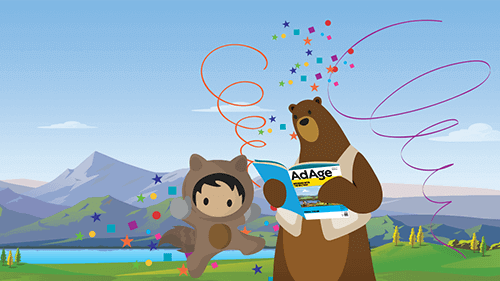
Using uiObjectInfoApi – get sObject metadata and RecordType Info
Using uiObjectInfoApi
api will allow us to retrieve the available metadata about a specific object. Like object label, fields, recordtypes etc… all that and without writing a single line of APEX.
How to use:
- Import
getObjectInfo
method. - Pass the object api name as a parameter or import the object name from the schema.
Example:
import { LightningElement, wire } from 'lwc'; import { getObjectInfo } from 'lightning/uiObjectInfoApi'; import ACCOUNT_OBJECT from '@salesforce/schema/Account'; export default class Example extends LightningElement { @wire(getObjectInfo, { objectApiName: ACCOUNT_OBJECT }) accountInfo; }
Pulling the ACCOUNT_OBJECT
using the @salesforce/schema
will provide compile-time safety to ensure the sObject is available on the schema. But, we are allowed to replaced it with a String for dynamic purposes.
Response:
{ "data": { "apiName": "Account", "childRelationships": [ { "childObjectApiName": "Account", "fieldName": "ParentId", "junctionIdListNames": [], "junctionReferenceTo": [], "relationshipName": "ChildAccounts" }, ... ], "createable": true, "custom": false, "defaultRecordTypeId": "012000000000000AAA", "deletable": true, "dependentFields": {}, "feedEnabled": true, "fields": { "Name": { "apiName": "Name", "calculated": false, "compound": true, "compoundComponentName": null, "compoundFieldName": "Name", "controllerName": null, "controllingFields": [], "createable": true, "custom": false, "dataType": "String", "extraTypeInfo": "SwitchablePersonName", "filterable": true, "filteredLookupInfo": null, "highScaleNumber": false, "htmlFormatted": false, "inlineHelpText": null, "label": "Account Name", "length": 255, "nameField": true, "polymorphicForeignKey": false, "precision": 0, "reference": false, "referenceTargetField": null, "referenceToInfos": [], "relationshipName": null, "required": true, "scale": 0, "searchPrefilterable": false, "sortable": true, "unique": false, "updateable": true }, ... }, "keyPrefix": "001", "label": "Account", "labelPlural": "Accounts", "layoutable": true, "mruEnabled": true, "nameFields": [ "Name" ], "queryable": true, "recordTypeInfos": { "012000000000000AAA": { "available": true, "defaultRecordTypeMapping": true, "master": true, "name": "Master", "recordTypeId": "012000000000000AAA" } }, "searchable": true, "themeInfo": { "color": "7F8DE1", "iconUrl": "https://ability-java-7718-dev-ed.cs26.my.salesforce.com/img/icon/t4v35/standard/account_120.png" }, "updateable": true } }
The Call will return the following result data – I have removed all fields and child relationship from this json for brevity.
Once we have the response we can access and get any metadata value we need…
Common use cases:
Get Record Type Ids
// GET DEFAULT RECORD TYPE ID get defaultRecordTypeId() { return this.accountInfo.data.defaultRecordTypeId; } // GET DEFAULT RECORD TYPE BY NAME fetchRecordTypeIdByName(recordTypeName) { // Returns a map of record type Ids const rtis = this.objectInfo.data.recordTypeInfos; return Object.keys(rtis).find(rti => rtis[rti].name === recordTypeName); }
Get Child Relationships from a parent object
get childReletionshipsInfo(){ return this.accountInfo.data.childRelationships; }
Get Object Label and Plural Label
// label get objectLabel(){ return this.accountInfo.data.label; } // plural label get objectPluralLabel(){ return this.accountInfo.data.labelPlural; }
Resources:
- getObjectInfo – Documentation