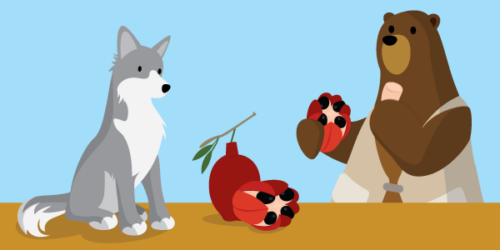
Using Custom Labels in Lightning Components
Beyond the common designed use case of Custom Labels that allows translation of labels into many languages.
I find them useful to allow me some placeholders functionality when needed.
What is Placeholder functionality in the context of Custom Labels ?
- If an admin user wish to change certain labels in the app to it’s own terminology – he can do so by changing the value of the Custom label and NOT go into the code of the component.
- Dynamically inject variables into a Fixed “placeholder” text. eg.
Range Between {0} to {1}
. - Custom Labels does not come instead of using other tools for “placeholder” functionality such as Custom Settings and Custom Metadata types.
Create Custom Labels
-
- Custom Labels live inside a metadata folder called
labels
- File name is
CustomLabels.labels-meta.xml
- Deploy to your org:
sfdx force:source:deploy -m CustomLabels -u <<ORGNAME>>
- Custom Labels live inside a metadata folder called
<?xml version="1.0" encoding="UTF-8"?> <CustomLabels xmlns="http://soap.sforce.com/2006/04/metadata"> <labels> <fullName>Title</fullName> <categories>General</categories> <language>en_US</language> <protected>false</protected> <shortDescription>Title</shortDescription> <value>Title</value> </labels> </CustomLabels>
- The above will add a new Custom label called
Title
Access Custom Labels
LWC
In Lightning Web Component we can do the following :
Import labels from the @salesforce/label
scoped module and declare them as properties.
- We must use a namespace as prefix => use
c.
for general custom namespace.
import { LightningElement } from 'lwc'; import labelTitle from '@salesforce/label/c.Title'; import labelPlaceholder from '@salesforce/label/c.Placeholder'; // value: 'WELCOME {0}' export default class CustomLabelComponent extends LightningElement { // CUSTOM LABELS label = { labelTitle, labelPlaceholder, ... } }
Using a private object variable named label
will allow us to mentain a certain convention for referencing our labels inside the HTML
<lightning-card title={label.labelTitle} > <div class="slds-p-around_small"> <p class="slds-text-heading_large"> {formattedPlaceholder} </p> </div> </lightning-card>
For Formatting or Dynamic populate values on the Custom Label – we can export and use a custom function
// display Write HELLO WORLD ! get formattedPlaceholder() { return this.format(labelPlaceholder, 'HELLO', 'WORLD'); } // eslint-disable-next-line no-unused-vars format(stringToFormat, ...formattingArguments) { if (typeof stringToFormat !== 'string') throw new Error('\'stringToFormat\' must be a String'); return stringToFormat.replace(/{(\d+)}/gm, (match, index) => (formattingArguments[index] === undefined ? '' : `${formattingArguments[index]}`)); }
Credit for this format()
method goes to this great example shared here
APEX
- Using Custom Labels in Apex
String s2 = System.Label.MyLabelName;
AURA
In Aura Component we could do the following :
const staticLabel = $A.get("$Label.c.task_mode_today");
– Luckily here we have a built-in function for format()
and we can access the custom label from the HTML markup and format the label with our placeholders attributes.
{!format($Label.mySection.myLabel, v.attribute1, v.attribute2)}